Microsoft Blazor Development: How to Build Interactive Web Apps with C#
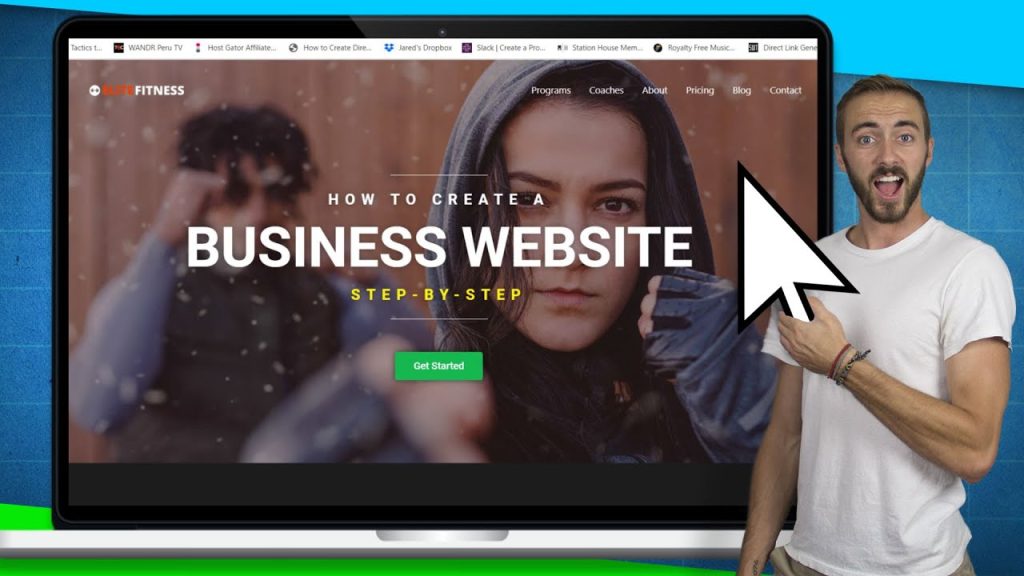
Web development has come a long way since its inception, and the demand for interactive web applications is on the rise. Microsoft Blazor is a web application framework that allows developers to create interactive web applications using C# instead of JavaScript. In this article, we’ll explore the power of Microsoft Blazor development, its fundamentals, and how to build interactive web apps using C#.
The Power of Microsoft Blazor Development
Microsoft Blazor is a game-changer in the world of web application development. It allows developers to create interactive web applications using C#, which is a popular language among developers. With Blazor, developers can now build a web application using a single language from the server to the client with the .NET runtime. It also gives developers full control over the client-side rendering of the web application.
The Future of Interactive Web Apps
Interactive web apps are the future of web development, and Blazor is leading the way. Blazor allows developers to create web applications that are highly interactive, without the use of JavaScript. This eliminates the need for developers to learn multiple languages, making the development process more efficient.
The Fundamentals of Microsoft Blazor
What is Microsoft Blazor?
Microsoft Blazor is an open-source web application framework that allows developers to build interactive web applications using C#. It was first released in 2018 and has since gained popularity among developers.
Blazor Web Assembly vs Blazor Server
There are two types of Blazor applications: Blazor Web Assembly and Blazor Server. Blazor Web Assembly is a client-side web application, and Blazor Server is a server-side web application. Blazor Web Assembly runs on the client’s browser, and Blazor Server runs on the server.
Getting Started with Microsoft Blazor Development
Building Components with C#
Blazor uses components to build web applications. Components are reusable pieces of code that can be shared across different parts of the application. Components can be built using C#.
C# Syntax and Classes
C# is a powerful language used to build components in Blazor. It has a rich set of features that allows developers to build complex components with ease. Classes in C# are used to define objects, which can then be used to build components.
Razor Markup and Components
Razor is a syntax used by Blazor to build components. It is similar to HTML but allows developers to write C# code within the markup. Razor also supports components, which can be used to build more complex components.
Rendering Components
Rendering components is the process of taking the component code and rendering it on the client-side. Blazor handles the rendering process automatically.
Managing State with Blazor
The State of a Component
The state of a component refers to the data that it holds. In Blazor, components can have their own state, which is managed by Blazor.
The Lifecycle of Components
Components have a lifecycle that they go through during their creation, modification, and disposal. Blazor manages the component lifecycle automatically.
State Management Techniques
Blazor provides several techniques for managing state, including component state, cascading values, and Flux/Redux patterns.
Navigation and Routing
The Importance of Navigation
Navigation is the process of moving between different pages or components within a web application. Navigation is a crucial part of any web application.
Setting up Routing
Routing allows developers to define the routes within the application. Blazor provides a powerful routing system that’s easy to use.
Navigating through a Blazor Application
Navigating through a Blazor application is similar to navigating through a traditional web application. Developers can use built-in methods to navigate between components.
Integrating External Libraries
Adding JavaScript Libraries to Blazor
Blazor allows developers to add JavaScript libraries to their web applications. This is done through a process known as interop.
Interacting with JavaScript from C#
Interop allows developers to bridge the gap between C# and JavaScript. This means that developers can call JavaScript code from C# and vice versa.
Enhancing User Experience with External Libraries
External libraries can be used to enhance the user experience of a web application. Blazor provides a simple way to integrate external libraries.
Debugging and Diagnostics
Debugging a Blazor Application
Debugging a Blazor application is similar to debugging any other .NET application. The Visual Studio debugger can be used to debug Blazor applications.
Issue Diagnosis
Diagnosing issues in a web application can be challenging. Blazor provides several built-in tools to help diagnose issues.
Common Debugging Strategies
Debugging a web application requires a different set of strategies than debugging traditional applications. Blazor provides several strategies for debugging web applications.
Optimization and Performance
Techniques for Optimal Performance
Blazor is designed to be performant out of the box. However, there are several techniques that developers can use to optimize the performance of their web applications.
Reducing Memory Footprint
Memory management is important in any application. Blazor provides several techniques for reducing the memory footprint of web applications.
Improving Load Times
Load times are a critical aspect of user experience. Blazor provides several techniques for improving the load times of web applications.
Deployment and Hosting
Deploying a Blazor Application
Deploying a Blazor application is similar to deploying any other .NET application. Developers can deploy their applications to different hosting providers, such as Azure.
Hosting Strategies
Blazor can be hosted on a server or a client. Developers can choose to host their applications in different ways depending on their requirements.
Best Practices for Deployment
Deploying a web application requires careful planning and execution. Blazor provides several best practices for deploying web applications.
Security Considerations
Security Threats and Vulnerabilities
Web applications are vulnerable to several security threats, such as cross-site scripting and SQL injection attacks. Blazor provides several built-in security features to mitigate these threats.
Implementing Security with Blazor
Blazor provides several features that can be used to implement security in web applications. This includes authorization, authentication, and data protection.
Authentication and Authorization
Authentication and authorization are essential components of any web application. Blazor provides several tools for implementing these features.
Cross-Platform Development
The Benefits of Cross-Platform Development
Cross-platform development allows developers to build web applications that can run on different platforms, such as desktop and mobile devices. Blazor provides several features for building cross-platform web applications.
Developing Mobile Applications with Blazor
Blazor can be used to build mobile applications using Xamarin. This allows developers to build mobile applications using C#.
Compatibility Considerations
Building cross-platform web applications requires careful consideration of compatibility issues. Blazor provides several features to mitigate these issues.
Applying Advanced Concepts
Integrating SignalR
SignalR is a real-time communication library that can be used to build real-time web applications. Blazor provides built-in support for SignalR.
Creating Custom Components
Custom components can be built using Blazor. This allows developers to build complex web applications with ease.
Working with Third-Party Libraries
Blazor can be used with third-party libraries to add additional functionality to web applications. This includes libraries for charting and mapping.
Best Practices for Blazor Application Development
Writing Readable Code
Readable code is essential for maintaining web applications. Blazor provides several features for writing readable code, such as component hierarchy and data binding.
Standardizing Development Processes
Standardizing development processes helps ensure consistency across web applications. Blazor provides several features for standardizing development processes.
Streamlining Testing
Testing is a critical part of web application development. Blazor provides several features for streamlining the testing process.
Blazor Resources and Communities
Getting Started Tutorials
Getting started tutorials can help developers get up to speed with Blazor quickly. There are several Blazor tutorials available online.
Official Microsoft Documentation
Microsoft provides official documentation for Blazor, which includes tutorials and documentation for different features.
Online Communities
Online communities are a great place to connect with other Blazor developers. There are several online communities dedicated to Blazor.
Challenges of Blazor Development and How to Overcome Them
Managing Complexity
Web applications can be complex, and managing complexity is essential to building and maintaining them. Blazor provides several features for managing complexity, such as component lifecycle management.
Ensuring Scalability
Web applications must be scalable to meet the demands of users. Blazor provides several features for ensuring scalability, such as server-side rendering.
Dealing with Compatibility Issues
Compatibility issues can arise when building cross-platform web applications. Blazor provides several features for mitigating these issues, such as platform-specific components.
Blazor vs. Other Web Development Frameworks
Comparing Blazor to Angular
Angular is a popular web development framework that uses TypeScript. Blazor and Angular have several similarities, but Blazor allows developers to build web applications using C#.
Blazor vs React
React is another popular web development framework that uses JavaScript. Blazor and React have several similarities, but Blazor allows developers to build web applications using C#.
Choosing the Right Framework
Choosing the right web development framework depends on several factors, such as developer experience and project requirements.
Frequently Asked Questions
What is Blazor used for?
Blazor is used for building interactive web applications using C#.
Can I use Blazor with JavaScript?
Blazor can work with JavaScript through a process known as interop.
Is Blazor SEO-friendly?
Blazor is SEO-friendly because it provides server-side rendering.
Conclusion
Microsoft Blazor is a powerful web application framework that allows developers to build interactive web applications using C#. It provides several features for managing state, optimizing performance, and building cross-platform web applications. Blazor is a game-changer in the world of web development, and developers should start exploring and developing with it.
--------------------------------
Guestposted.com Notice!
Audience discretion is needed, Read TOS.
Submit Guest Post / Read Latest / Category List
App & Rate-Us / Subscribe Daily Newsletter (FREE)
-
A tech nerd from heart, and marketing & lead generation expert by profession, He shares valuable insight into technology & business trends through his passion for writing.
- https://en.gravatar.com/leadmustermarketing